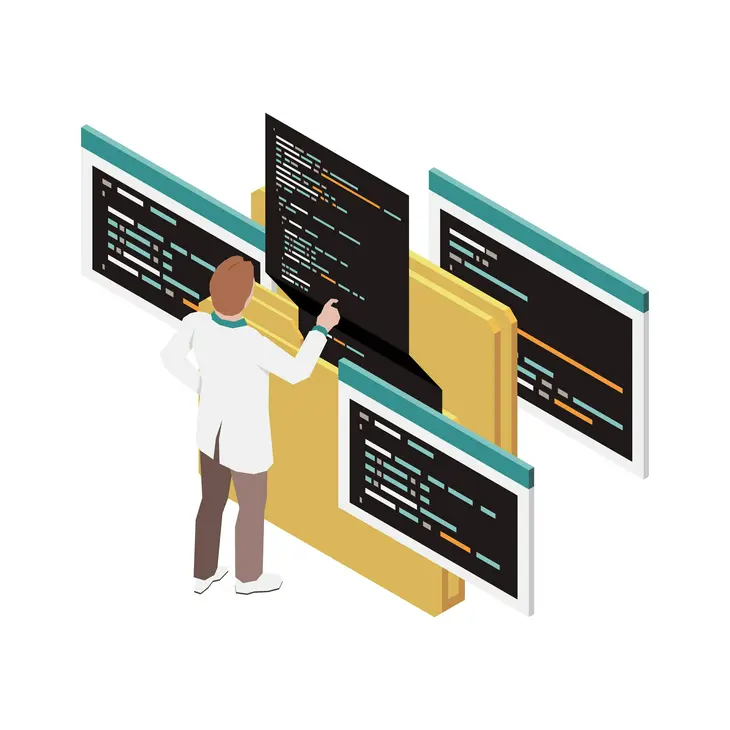
Title: Exploring Advanced Swift Features: Beyond the Basics
- Admin
Title: Exploring Advanced Swift Features: Beyond the Basics
Congratulations on mastering the fundamentals of Swift programming! Now that you're comfortable with the basics, it's time to explore some of the more advanced features that Swift has to offer. In this article, we'll delve into advanced Swift concepts and techniques that will take your coding skills to the next level.
1. Generics
Generics allow you to write flexible, reusable code by defining functions, structures, and classes that can work with any type. Learn how to use generics to write more generic and type-safe code, avoiding unnecessary duplication and improving the flexibility of your Swift programs.
2. Protocols and Protocol-Oriented Programming (POP)
Protocols are powerful tools in Swift for defining blueprints of methods, properties, and other requirements that types can conform to. Explore how protocols can be used to achieve code reuse, composition, and polymorphism, and discover the principles of Protocol-Oriented Programming (POP) for building flexible and modular codebases.
3. Advanced Error Handling
Go beyond the basics of error handling and explore advanced techniques for managing errors in your Swift code. Learn about custom error types, throwing and catching errors in asynchronous code, and leveraging Result types for more expressive error handling.
4. Property Wrappers
Property wrappers are a powerful feature introduced in Swift 5.1 that allow you to add custom behavior to properties. Discover how property wrappers can be used to encapsulate common property patterns, such as validation, lazy initialization, and data transformation, leading to cleaner and more concise code.
5. Memory Management and ARC
Swift uses Automatic Reference Counting (ARC) to manage memory allocation and deallocation automatically. Dive deeper into memory management in Swift, including understanding strong, weak, and unowned references, managing reference cycles, and optimizing memory usage in your Swift applications.
6. Advanced Functional Programming Techniques
Functional programming is at the core of Swift's design philosophy, and there are many advanced techniques and patterns that you can leverage to write more expressive and concise code. Explore topics such as higher-order functions, currying, function composition, and monads, and learn how to apply them effectively in your Swift projects.
7. Concurrency and Parallelism
Concurrency and parallelism are essential concepts for building responsive and scalable applications. Discover advanced techniques for concurrent and parallel programming in Swift, including DispatchQueue, OperationQueue, async/await, and Combine framework, and learn how to write efficient and thread-safe code in Swift.
8. Advanced Swift Debugging and Optimization
Learn advanced debugging and optimization techniques to diagnose and fix performance issues in your Swift code. Explore tools such as Instruments, LLDB debugger, and XCTest framework for profiling, debugging, and testing your Swift applications, and optimize your code for speed, memory usage, and battery efficiency.
By mastering these advanced Swift features and techniques, you'll be equipped to tackle more complex programming challenges and build even more sophisticated and scalable iOS, macOS, watchOS, and tvOS applications. Keep exploring, experimenting, and pushing the boundaries of what's possible with Swift!
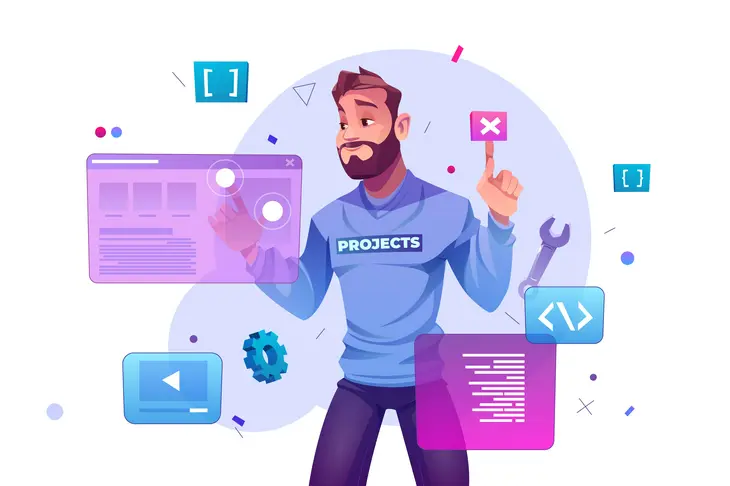